Introduction: Laravel has emerged as one of the most popular PHP frameworks for web application development. It offers a wide range of features and tools that streamline the development process. However, like any other technology, Laravel is not immune to common mistakes and anti-patterns that can impact the performance, security, and maintainability of applications. In this article, we will explore some of the common anti-patterns in Laravel development and discuss strategies to avoid them.
I. Improper Database Usage:
A. Lack of Database Abstraction: Laravel provides a powerful database abstraction layer called Eloquent. Unfortunately, some developers still resort to writing raw SQL queries instead of leveraging the benefits of Eloquent. This approach not only hampers code readability but also introduces security risks and reduces portability. Eloquent provides a convenient and expressive way to interact with the database. Instead of writing raw SQL queries, you can define models that represent database tables and use Eloquent's query builder methods. For example:
$users = User::where('status', 'active')->get();
In the above example, we use the User model to query the database for users with an 'active' status. This approach not only improves code readability but also ensures that the query is properly escaped, protecting against SQL injection attacks.
B. N+1 Query Problem: The N+1 query problem occurs when a query is executed to retrieve a set of records and an additional query is executed for each record to fetch related data. This can lead to a significant performance bottleneck. Laravel addresses this problem through eager loading relationships. By specifying the relationships to be loaded upfront, you can minimize the number of queries. For example, consider the following scenario:
$posts = Post::with('comments')->get();
In this example, we use the with() method to eager load the comments relationship for the Post model. Without eager loading, retrieving comments for each post would require an additional query for each post, resulting in the N+1 query problem. Eager loading ensures that all the necessary data is fetched in a single query, significantly improving performance.
C. Missing Indexes: Database indexes play a crucial role in optimizing query performance. Failure to properly index the database tables can result in slow queries, especially when dealing with large datasets. Regularly analyze your query patterns and identify the columns frequently used in the WHERE and JOIN clauses. By adding appropriate indexes to these columns, you can drastically improve query speed. For example, let's say you have a users table with a column named email that is frequently used in search queries. You can add an index to the email column using Laravel's migration feature:
Schema::table('users', function (Blueprint $table) {
$table->index('email');
});
Adding an index to the email column can significantly improve the performance of queries that involve searching for users by their email addresses.
II. Inefficient Queries:
A. Selecting All Columns: Fetching all columns in a query, especially when only a few are needed, can impact performance. It increases the amount of data transferred between the database and the application, leading to slower execution. To optimize queries, specify only the required columns in your queries. For instance:
$users = User::select('name', 'email')->get();
In the above example, we select only the name and email columns from the users table. This reduces the amount of data retrieved from the database, resulting in faster and more efficient queries.
B. Excessive Eager Loading: While eager loading can improve performance, loading unnecessary relationships can have the opposite effect. Carefully evaluate the relationships you need to load and avoid overloading your models with eager loading. Instead, use lazy loading or explicit eager loading when appropriate to avoid unnecessary data retrieval. Lazy loading allows you to load relationships only when they are accessed. For example:
$posts = Post::all();
foreach ($posts as $post) {
// Lazy load the comments relationship
$comments = $post->comments;
}
In this example, the comments relationship is loaded only when accessed within the loop. This ensures that the relationship data is retrieved only when needed, reducing unnecessary database queries.
Explicit eager loading is another approach that allows you to specify the relationships to be loaded. For example:
$posts = Post::with('comments')->get();
By explicitly stating the relationships to be loaded, you have more control over which relationships are fetched, avoiding excessive eager loading.
C. Improper Pagination: When dealing with large result sets, it's essential to implement pagination. Fetching and displaying all records at once can put a strain on server resources and impact the user experience. Laravel provides convenient pagination features that allow you to limit the number of records fetched. For example:
$posts = Post::paginate(10);
In the above example, the paginate() method retrieves 10 records at a time, making it easier to navigate through the results. This improves performance by reducing the amount of data retrieved from the database and enhances the user experience by providing a more manageable and responsive interface.
III. Poor Error Handling:
A. Lack of Error Logging: Error logging is crucial for effective debugging and issue resolution. Laravel offers built-in logging mechanisms that simplify error tracking. By configuring logging in your Laravel application, you can easily monitor and analyze errors, making troubleshooting more efficient. Laravel provides various logging channels, including file logging and database logging. By specifying the desired logging channel and configuring the log level, you can ensure that errors are captured and logged appropriately.
B. Insufficient Validation: Inadequate input validation exposes your application to security vulnerabilities and data integrity issues. Laravel's validation features provide a robust solution to validate user input. Leverage Laravel's validation rules and error messages to ensure that data is validated properly before further processing. For example, let's say you have a form where users can submit their email addresses. You can validate the email address using Laravel's validation rules:
$request->validate([
'email' => 'required|email',
]);
In the above example, we validate the email field, ensuring that it is required and in the correct email format. If the validation fails, Laravel automatically redirects the user back to the form with appropriate error messages.
C. Generic Error Messages: Generic error messages can confuse and frustrate users. Instead, strive to provide specific and user-friendly error messages that guide users towards resolving the issue. By customizing error messages based on the context and providing clear instructions, you can improve the user experience and aid in issue resolution. Laravel allows you to define custom error messages for each validation rule. For example:
$request->validate([
'email' => 'required|email',
], [
'email.required' => 'Please provide your email address.',
'email.email' => 'Please enter a valid email address.',
]);
In the above example, we customize the error messages for the required and email validation rules, providing clear instructions to the user.
Conclusion: In the world of Laravel development, avoiding common mistakes and anti-patterns is crucial to ensure efficient, secure, and maintainable applications. By adhering to best practices, such as leveraging Eloquent for database interactions, optimizing queries, and implementing robust error handling, developers can enhance their Laravel applications' performance and user experience. Stay vigilant, continuously learn, and embrace Laravel's conventions to develop high-quality applications.
If you're a Laravel enthusiast seeking to expand your knowledge and refine your skills, check our blog post on The most powerful Laravel/PHP tool you're not using yet
Expand your knowledge even further by exploring these insightful articles related to the topic:
https://martinjoo.dev/common-mistakes-in-laravel
https://laraveldaily.com/post/laravel-typical-mistakes-juniors-make
https://devpark.pl/5-of-the-common-mistakes-made-by-laravel-programmers/
"Only those who dare to fail greatly can ever achieve greatly."
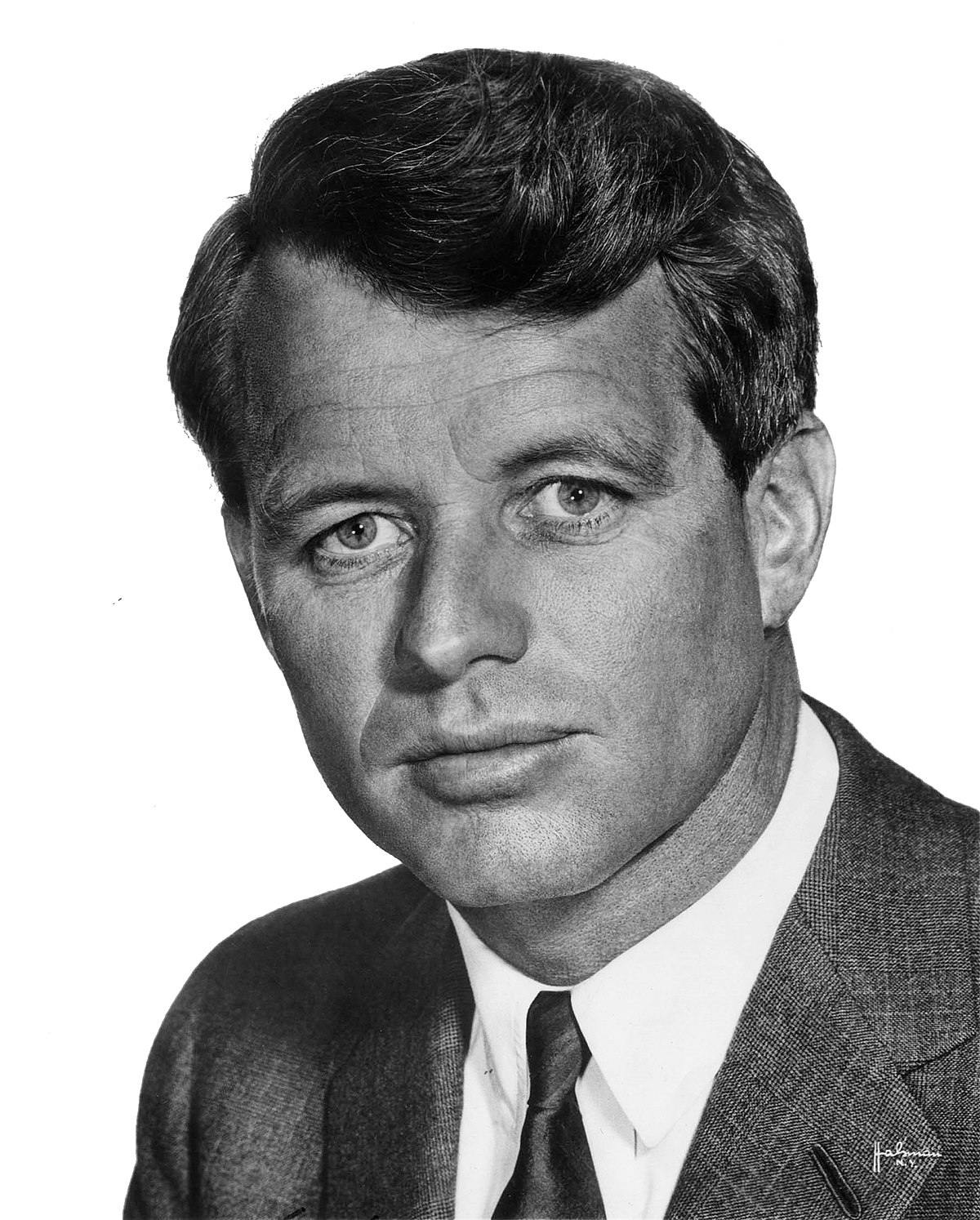