Introduction
This blog post is tailored for both technical professionals, such as developers and software engineers, seeking to leverage feature flagging techniques to optimize their development workflow. Additionally, it is equally relevant for non-technical specialists, like marketing professionals, who aim to gain insights into how feature flagging can enhance A/B testing, user engagement, and business strategies.
Throughout this article, we will delve into feature flagging's implementation within Laravel, a widely adopted PHP framework, and thoroughly explore its vast array of benefits, including gradual rollouts, A/B testing capabilities, risk mitigation, and harmonious integration with continuous deployment pipelines.
I. Understanding Feature Flagging
A. Definition and Purpose
- Feature flagging: a technique to control feature availability and behavior in applications.
- Purpose: enable gradual rollouts, A/B testing, risk mitigation, and more.
B. Benefits of Feature Flagging in Laravel
1. Controlled Rollouts:
- Gradual feature releases: minimize risks by exposing new features to a subset of users.
- Canary releases: monitor feature performance in production with a small audience.
2. A/B Testing and Experimentation:
- Evaluate the impact of different feature variations on user behavior and business metrics.
- Optimize user experience and conversions through data-driven decisions.
3. Risk Mitigation:
- Safeguard against unforeseen issues by easily disabling or rolling back features.
- Avoid impacting users or business operations during critical situations.
4. Continuous Integration and Deployment:
- Seamlessly integrate feature flagging with CI/CD pipelines.
- Simplify deployment processes by decoupling feature releases from code deployments.
- Allows to control backend and frontend deployment simultaneously.
II. Best Practices for Effective Feature Flagging
A. Clear Documentation and Communication
- Document feature flag names, their purpose, and expected behavior.
- Communicate feature flag changes to the development team and stakeholders.
B. Monitoring and Metrics
- Implement logging and monitoring to track feature usage and performance.
- Capture user feedback and behavior metrics to evaluate feature impact.
C. Feature Flag Lifecycles
- Establish a plan for removing obsolete feature flags to maintain code cleanliness.
- Define criteria for promoting flags from development to production.
D. Testing and Quality Assurance
- Thoroughly test different feature flag scenarios to ensure expected behavior.
- Conduct QA checks specifically focused on flagged features.
III. Implementing Feature Flagging in Frontend Development
In the context of frontend development, the implementation of feature flagging involves integrating the feature flags into the user interface and controlling the visibility and behavior of specific features based on their flag states. Here's a brief explanation of how the concepts discussed in the blog post would be implemented on the frontend:
A. Controlled Rollouts:
In the frontend, feature flags can be used to conditionally render UI components or sections based on the flag states.
By checking the flag status, developers can gradually expose new features to a subset of users, allowing for controlled rollouts.
The visibility of new UI elements or interactions can be selectively enabled for specific user segments, gradually expanding the feature's reach.
B. A/B Testing and Experimentation:
Feature flags allow frontend developers to conditionally render different variations of UI elements, styles, or interactions.
By assigning users to different feature flag groups, A/B testing can be performed to compare the effectiveness of different UI variations.
Developers can collect user feedback and behavioral metrics to evaluate the impact of different UI experiences on user engagement and conversions.
C. Risk Mitigation:
If issues are identified in the frontend, feature flags provide a mechanism to quickly disable or roll back specific features.
By toggling the flag states, developers can instantly remove or modify problematic UI components, reducing the impact on users and minimizing risks.
This allows for swift mitigation of frontend issues without requiring a full deployment or code rollback.
D. Continuous Integration and Deployment:
Frontend feature flags can be seamlessly integrated into the CI/CD pipelines to automate the process of enabling or disabling features.
Through proper configuration and deployment practices, frontend code changes related to feature flags can be deployed independently from the rest of the application.
This decoupling of feature releases from code deployments simplifies the deployment process and enables faster iteration and feature experimentation.
IV. Feature Flagging in the context of Laravel Nova
In the context of Laravel Nova, a powerful administration panel for Laravel applications, feature flagging can be seamlessly integrated to control the visibility and behavior of specific features within the admin interface. Here are a few examples of how feature flagging would look like in Nova, complementing the concepts discussed in the article:
A. Controlled Rollouts:
Within Nova, feature flags can be used to gradually release new administrative features to a subset of users or administrators.
By conditionally displaying menu items or custom tools based on the flag states, developers can expose new functionality to specific user roles or groups.
This allows for controlled rollouts, where administrators can gain early access to new features, ensuring a smoother transition and minimizing potential disruptions. QA teams can benefit from this features a lot, too.
B. A/B Testing and Experimentation:
Feature flags in Nova can be utilized to test different variations of administrative workflows, layouts, or custom tools.
By conditionally rendering alternative components or views, developers can create A/B testing scenarios within the administration panel.
This empowers administrators to provide feedback and insights on different variations, allowing for data-driven decisions to optimize the usability and effectiveness of the admin interface.
C. Risk Mitigation:
In case of issues or unexpected behavior in Nova, feature flags can serve as a safety net by allowing developers to quickly disable or modify specific administrative features.
By toggling the flag states, problematic or unstable components can be temporarily hidden from administrators, ensuring uninterrupted access to critical functionality.
This ensures that any potential issues or bugs in the admin interface can be promptly addressed without impacting the overall functionality of the application.
D. Continuous Integration and Deployment:
Nova feature flags can seamlessly integrate with the CI/CD pipeline, allowing for independent feature releases within the administration panel.
By incorporating feature flags into the deployment process, developers can enable or disable administrative features without requiring a full application deployment.
This decoupling of feature releases from the main application deployment simplifies the release management process for Nova, ensuring a smoother and more efficient workflow.
Here are a few practical examples of how feature flagging can be implemented in Laravel Nova with code snippets:
Controlled Rollouts:
// In your NovaServiceProvider, define a feature flag for a new feature
use Laravel\Nova\Nova;
Nova::serving(function () {
if (Feature::isEnabled('new_feature')) {
// Register the new feature resources
Nova::resources([
// Add new feature resources here
]);
}
});
In the above example, the "new_feature" flag is checked, and if it is enabled, the corresponding resources for the new feature are registered within Laravel Nova. This ensures that the new feature is only visible and accessible to users when the flag is enabled.
A/B Testing and Experimentation:
// In your Nova resource file, conditionally render different UI variations
public function fields(Request $request)
{
if (Feature::isEnabled('variation_a')) {
return [
// Fields for variation A
];
} elseif (Feature::isEnabled('variation_b')) {
return [
// Fields for variation B
];
} else {
return [
// Default fields
];
}
}
In this example, within a Nova resource file, different variations of UI fields are conditionally rendered based on the feature flags. Depending on the enabled flag, either variation A or variation B fields are displayed to the administrators, allowing for A/B testing and experimentation.
Risk Mitigation:
// In your Nova resource file, disable a problematic field or panel
public function fields(Request $request)
{
return [
// Other fields
Feature::isEnabled('problematic_field')
? Text::make('Problematic Field', 'problematic_field')
: Text::make('Backup Field', 'backup_field'),
// Other fields
];
}
In this example, a potentially problematic field is conditionally rendered based on the feature flag state. If the "problematic_field" flag is enabled, the field is displayed. However, if the flag is disabled, a backup field is rendered instead. This allows developers to quickly mitigate issues by replacing or hiding problematic features in Nova.
Similarly with Nova, you can expose feature flags using Laravel Restify via API so frontend can benefit from it. Laravel Restify is an open-source package from BinarCode. It offers a straightforward way to create JSON APIs with features like advanced searching, sorting, and pagination built-in. Contribute, learn, and improve your workflow with Laravel Restify on GitHub. Embrace the faster, streamlined API development it offers.
Conclusion:
Feature flagging emerges as a powerful and versatile technique that not only empowers Laravel developers but also caters to the needs of marketing specialists within the tech industry. Both Laravel Nova and Laravel Restify provide exceptional platforms for seamless feature flagging integration, offering developers and non-technical professionals alike the opportunity to harness the full potential of this approach.
For developers, feature flagging in Laravel projects ensures precise control over feature availability, effective risk management, and streamlined deployments. With the ability to easily manage and toggle feature visibility, developers can confidently experiment and iterate on new functionalities, delivering enhanced user experiences.
Equally significant for marketing specialists, feature flagging serves as a strategic asset for A/B testing, enabling data-driven decision-making and targeted user engagement. By leveraging feature flagging within Laravel projects, marketing professionals can optimize promotional strategies, refine user experiences, and adapt their campaigns for maximum impact.
Progress is impossible without change, and those who cannot change their minds cannot change anything.
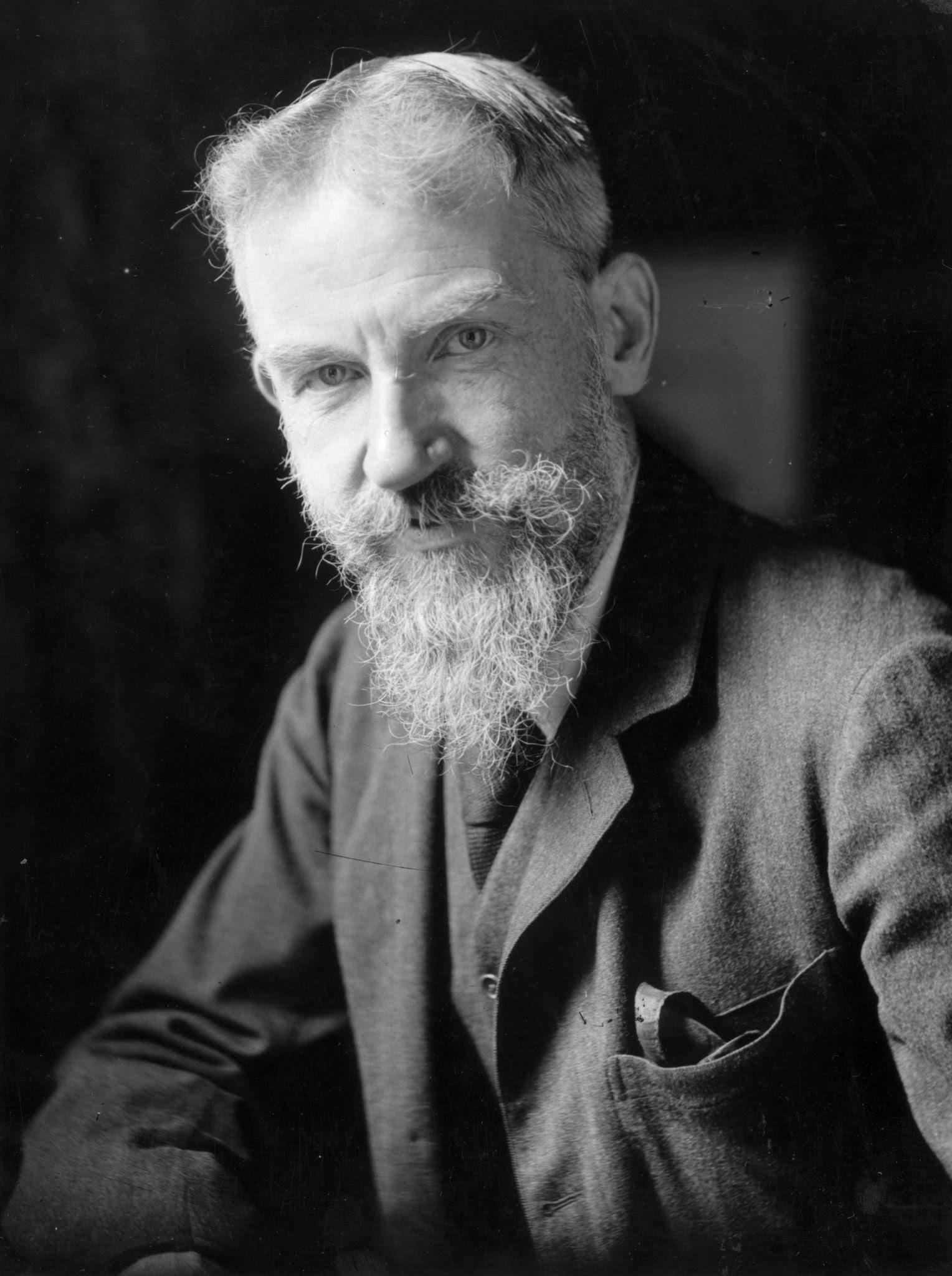